18
Legacy C Library Headers>> cassert | cctype | cerrno | cfloat | ciso646 | climits | clocale | cmath | csetjmp | csignal | cstdarg | cstddef | cstdio | cstdlib | cstring | ctime | cwchar | cwctype 20 Specifically C++ Library
Headers>> [10 stream headers>>] fstream | iomanip | ios | iosfwd | iostream | istream | ostream | sstream | streambuf | strstream
[4 language support
headers>>] exception | new | stdexcept | typeinfo [6 odds and ends headers>>] bitset | complex | limits | locale |
string |
valarray 13 STL Library Headers
This C++ Standard Library reference is abbreviated to contain only
the most frequently used items. It implicitly assumes a Standard ASCII
world (so there is no wide-character material), and it will probably be
most useful to C++ programming students at "western" universities.
The information included here is not meant to be complete or
definitive in any way, but more of a quick reference or quick reminder
of what something looks like if you need to look it up in a hurry, and
you've left your handy hard-copy reference on the bus. Thus,
infrequently used functions (or variations of functions) and other
entities may not appear.
The page contains a list of all 51 C++ header files that are
currently (summer, 2007) either officially part of the C++ Standard
Library, or regarded as being associated very closely with it, in the
sense that they provide facilities that closely parallel those of the
"official" headers, and can be used in much the same way.
This header list includes the 18 headers containing the (revised
legacy) content from the C Standard Library, the 20 new "specifically
C++" headers, and the 13 (even newer) headers of the Standard Template
Library. The STL headers are given here for the sake of completeness,
but all detailed STL information available on this web site is
contained in other site pages
starting here. The current page also includes a selection of
some of the many data types, functions, and other entities
available from some of the (non-STL) headers. Although it may
not be strictly true, the average programmer is probably best served by
regarding everything in all of these headers as being contained in
namespace std.
As for the organization of this web page, note first that within any
given header grouping, the individual headers are listed in
alphabetical order. Under any given header, the entries may also be
arranged in alphabetical order, but more likely you will find items
(functions, for example) grouped in some other way (by related purpose,
or by historical tradition, say). (For example, under
<cmath> all the trig functions are grouped together, but
not in alphabetical order.) This decision represents a compromise, of
course, since an alphabetical listing provides quickest access to a
function if you know what you're looking for. However, this reference
page is meant just as much for browsing, to see what might be
available, and for that kind of use other groupings tend to be more
helpful.
Though full function prototypes are generally provided, in some
cases (manipulators for example, and functions with complex and
potentially confusing prototypes), it seemed more reasonable (and
useful) to provide the items in "typical usage" format. See the
string class, for example. This "typical usage" format will
often include parameter names that consist of the type name of the
parameter and a portion of the name to suggest parameter usage. For
example, size_type_count might be used as the name of a
parameter of type size_type that will contain a count of some
kind.
The 18 Legacy Headers from the Standard C Library
<cassert>
This header declares the assert() macro. The header is
unique in that it can be included multiple times to obtain different
effects, depending on whether the NDEBUG macro is defined at
the time of inclusion.
- void assert(int expression)
- This macro terminates a program if expression evaluates
to 0, by printing a message to standard error and calling
abort().
- NDEBUG
-
If this macro is defined (by the programmer) before
<cassert> is included, then subsequent calls to
assert() are disabled. A point of potential confusion here
is that "defining" NDEBUG does not require actually giving
it a value. So, it is OK to simply say
#define NDEBUG
<cctype>
This header declares several functions for dealing with ordinary
ASCII characters (or, more generally, "narrow" character types). Note
that, perhaps somewhat surprisingly, each function takes an
int parameter and returns an int value. However, the
actual parameter must be an unsigned char value, and though
implicit conversion may work most of the time, it is better (and safer)
programming practice to cast both the parameter and the return value to
the appropriate type.
- int isalnum(int ch)
- Return true (non-zero) if ch is alphanumeric
[equivalent to isalph(ch)
|| isnumeric(ch)]
- int isalpha(int ch)
- Return true (non-zero) if ch is alphabetic (an
uppercase or lowercase letter)
- int iscntrl(int ch)
- Return true (non-zero) if ch is a control
character (in 7-bit ASCII, a character with decimal code 127 or
decimal code lying in the range 0..31 [equivalent to !isprint(ch)]
- int isdigit(int ch)
- Return true (non-zero) if ch is a digit
character (a character in the range '0'..'9')
- int isgraph(int ch)
- Return true (non-zero) if ch is a non-blank
printable character (in 7-bit ASCII, any character in the range '!'..'~', i.e., any character with a
decimal code in the range 33..126)
- int islower(int ch)
- Return true (non-zero) if ch is a lowercase
letter (any character in the range 'a'..'z')
- int isprint(int ch)
- Return true (non-zero) if ch is a printable
character, including the blank space (in 7-bit ASCII, any character
with a decimal code lying in the range 32..126)
- int ispunct(int ch)
- Return true (non-zero) if ch is a punctuation
character (i.e., any printable character other than a blank space or
an alphanumeric character [equivalent to isgraph(ch) && !isalnum(ch)]
- int isspace(int ch)
- Return true (non-zero) if ch is a whitespace
character (blank space, newline ('\n'), horizontal tab
('\t'), vertical tab ('\v'), carriage return
('\r'), or form feed ('\f')) [Note that the
backspace character ('\b') is not included in this
list.]
- int isupper(int ch)
- Return true (non-zero) if ch is an uppercase
letter (any character in the range 'A'..'Z')
- int isxdigit(int ch)
- Return true (non-zero) if ch is a hexadecimal
digit character (any character lying in one of the ranges '0'-'9', 'a'-'f', or 'A'-'F')
- int tolower(int ch)
- Return ch converted to the corresponding lowercase
value, if this makes sense (i.e., if ch is already an
uppercase letter); otherwise return ch
- int toupper(int ch)
- Return ch converted to the corresponding uppercase
value, if this makes sense (i.e., if ch is already a
lowercase letter); otherwise return ch
<cerrno>
This header declares several macros related to error-handling in the
Standard Library.
<cfloat>
This header defines macros that characterize floating-point types in
the same way that <climits> does for the integral types.
The native C++ header <limits> defines the same information (and
more) using the (more modern) template approach instead of macros.
- int FLT_DIG
- Macro defining the number of significant digits in a
float value (always at least 6)
- float FLT_MAX
- Macro defining the maximum positive float value (always
at least 1037)
- float FLT_MIN
- Macro defining the minimum positive float value (always
at most 10-37)
- int DBL_DIG
- Macro defining the number of significant digits in a
double value (always at least 10)
- double DBL_MAX
- Macro defining the maximum positive double value (always
at least 1037)
- double DBL_MIN
- Macro defining the minimum positive double value (always
at most 10-37)
- int LDBL_DIG
- Macro defining the number of significant digits in a long
double value (always at least 10)
- long double LDBL_MAX
- Macro defining the maximum positive long double value
(always at least 1037)
- long double LDBL_MIN
- Macro defining the minimum positive long double value
(always at most 10-37)
<ciso646>
This header does nothing, and is present only for the sake of
completeness, since every header in the C Standard Library must have a
counterpart in C++.
<climits>
This header defines parameters that characterize integral types in
the same way that <cfloat> does for the floating-point
types. The native C++ header <limits> defines the same
information (and more) using the (more modern) template approach
instead of macros.
- int CHAR_BIT
- Macro defining the number of bits in a byte (always at
least 8)
- char CHAR_MAX
- Macro defining the maximum char value
- char CHAR_MIN
- Macro defining the minimum char value
- short SHRT_MAX
- Macro defining the maximum positive short int value
(always at least 32767)
- short SHRT_MIN
- Macro defining the minimum negative short int value
(always at most -32767)
- int INT_MAX
- Macro defining the maximum positive int value (always at
least 32767)
- int INT_MIN
- Macro defining the minimum negative int value (always at
most -32767)
- long int LONG_MAX
- Macro defining the maximum positive long int value
(always at least 2147483647)
- long int LONG_MIN
- Macro defining the minimum negative long int value
(always at most -2147483647)
- unsigned char UCHAR_MAX
- Macro defining the maximum unsigned char value (always
at least 255)
- unsigned short USHRT_MAX
- Macro defining the maximum unsigned short int value
(always at least 65535)
- unsigned int UINT_MAX
- Macro defining the maximum unsigned int value (always at
least 65535)
- unsigned long ULONG_MAX
- Macro defining the maximum unsigned long int value
(always at least 4294967295)
<clocale>
This header declares types and functions to support
internationalization and localization for the C++ Standard. So does the
newer header file <locale>, but at the cost of
additional complexity and overhead. Usually it is OK to live with your
system's defaults and ignore this header.
<cmath>
This header declares a number of mathematical functions. In addition
to the standard functions carried over from the C library, most of
these functions have overloaded versions for different parameter types,
and for each listed function all overloaded versions are shown.
- float abs(float x)
double abs(double x)
long double abs(long double x)
- Return the absolute value of x
float fabs(float x)
double fabs(double x)
long double fabs(long double x)
- Return the absolute value of the float (or
double, or long double) value x
- float sin(float x)
double sin(double x)
long double sin(long double x)
- Return the sine of x radians (always a value in
[-1,1])
float cos(float x)
double cos(double x)
long double cos(long double x)
- Return the cosine of x radians (always a value in
[-1,1])
float tan(float x)
double tan(double x)
long double tan(long double x)
- Return the tangent of x radians (the Standard does not
specify a return value if x has a value of the form kπ + π/2, k an integer)
float asin(float x)
double asin(double x)
long double asin(long double x)
- Return the inverse sine of x (x must be in
[-1,1] and the return value will be in the range [-π/2,
π/2])
float acos(float x)
double acos(double x)
long double acos(long double x)
- Return the inverse cosine of x (x must be in
[-1,1] and the return value will be in the range [0,π])
float atan(float x)
double atan(double x)
long double atan(long double x)
- Return the inverse tangent of x (return value will be in
the range (-π/2,π/2))
- float sinh(float x)
double sinh(double x)
long double sinh(long double x)
- Return the hyperbolic sine of x
float cosh(float x)
double cosh(double x)
long double cosh(long double x)
- Return the hyperbolic cosine of x
float tanh(float x)
double tanh(double x)
long double tanh(long double x)
- Return the hyperbolic tangent of x
- float exp(float x)
double exp(double x)
long double exp(long double x)
- Return e to the power of x (e is the value
2.71828...)
float log(float x)
double log(double x)
long double log(long double x)
- Return the logarithm of x to the base e
(e is the value 2.71828...) (i.e., the "natural logarithm"
of x)
float log10(float x)
double log10(double x)
long double log10(long double x)
- Return the logarithm of x to the base 10 (i.e., the
so-called "common logarithm" of x) [Note that x
must be > 0.]
- float ceil(float x)
double ceil(double x)
long double ceil(long double x)
- Return the ceiling of x (smallest integer >=
x)
float floor(float x)
double floor(double x)
long double floor(long double x)
- Return the floor of x (largest integer <= x)
(also called the "greatest integer function")
- float pow(float x, float y)
float pow(float x, int y)
double pow(double x, double y)
double pow(double x, int y)
long double pow(long double x, long double y)
long double pow(long double x, int y)
- Return base x raised to the power y (i.e.,
exponent y) [Note that if y is not an integer, then
x must be > 0.]
float sqrt(float x)
double sqrt(double x)
long double sqrt(long double x)
- Return the square root of x [Note that x must
be >= 0, and the return value will always be >= 0 as
well.]
<csetjmp>
This header generally has limited use in C++ programs. In C++,
exceptions should be used in place of the facilities the C counterpart
of this header supplies to C programs.
<csignal>
This header declares functions and macros related to signal
handling, where a signal is a condition that can arise
during program execution.
<cstdarg>
This header declares macros for accessing the arguments to a
function that takes a variable number of arguments. Such a function is
called a variadic function, and is indicated by having an
ellipsis (...) as its last parameter.
<cstddef>
This header defines several useful types and macros.
- NULL
- The NULL macro expands to a constant null pointer whose
value is implementation-dependent, but usually 0 or 0L.
- ptrdiff_t
- A typedef for an implementation-dependent signed
integral type which represents the difference between two
pointers
- size_t
- A typedef for an implementation-dependent signed
integral type which is the type returned by the sizeof
operator
<cstdio>
This is a large library, which declares many types, functions and
macros which deal with input and output, and which are compatible with
the C programming language. However, C++ I/O streams offer more
flexibility, type-safety and clarity. Moreover, certain problems can
occur when both the C <stdio> library and the C++
<iostream> library are used in the same program. It may
be inferred from the last statement, and rightly so, that it is just
not good programming practice to make simultaneous use of these two
libraries. In general, I/O in C++ programs should be performed using
C++ I/O facilities. Thus, to encourage this practice, we do not include
any of the items from this header in the current reference.
<cstdlib>
This header declares a number of generally useful macros, types and
functions.
- NULL
- The NULL macro expands to a constant null pointer whose
value is implementation-dependent, but usually 0 or 0L.
- size_t
- A typedef for an implementation-dependent signed
integral type which is the type returned by the sizeof
operator
- int EXIT_FAILURE
- Call exit(EXIT_FAILURE) to terminate a program normally
and inform the operating system that the program was unsuccessful.
[Returning EXIT_FAILURE from main is the same as calling
exit(EXIT_FAILURE).]
- int EXIT_SUCCESS
- Call exit(EXIT_SUCCESS) to terminate a program normally
and inform the operating system that the program was successful
[Returning EXIT_SUCCESS from main is the same as calling
exit(EXIT_SUCCESS).]
- void abort()
- Default action is simply to terminate a program, generally
without any "cleanup", and so acts as C++'s "emergency stop" function
[The exit() function should generally be preferred for
"sudden, but normal" termination.]
- int abs(int x)
long abs(long x)
- Return absolute value of int (or long) value
x
- long labs(long x)
- Return absolute value of long integer value x [This
function is clearly not required, given the overloaded version of the
abs() function (above), but is present for backward
compatibility with C.]
- double atof(const char* str)
- Return the double form of str
- int atoi(const char* str)
- Return the int form of str
- long atol(const char* str)
- Return the long int form of str
- div_t div(int numerator, int denominator)
-
Divide numerator by denominator and return both in a structure of
type div_t, which is defined as follows:
struct div_t { int quot, rem; };
- ldiv_t div(long numerator, long denominator)
-
Divide numerator by denominator and return both in a structure of
type ldiv_t, which is defined as follows:
struct ldiv_t { long quot, rem; };
- extern int atexit(void (*func)())
- Register a parameterless function func to execute at
(normal) program termination [Multiple functions can be registered,
and registered functions are called in the opposite order of
registration. A function can be registered more than once, and will
be called as many times as it was registered.]
- void exit(int status_code)
- Terminate program normally, with status_code indicating
"program status" [A status_code value of 0 is generally
taken to mean successful termination, with other return values being
implementation-dependent.]
- char* getenv(const char* var_name)
- Return the value of environment variable var_name
- RAND_MAX
- Maximum possible value of the rand() function
- int rand()
- Return a "pseudorandom" integer in the range 0..RAND_MAX
- void srand(unsigned int seed)
- Set the "seed value" for the function rand() [The
default seed value used for rand(), if this function is not
called, is 1.]
- int system(const char* com)
- Pass the command in the C-string com to the operating
system to be executed
<cstring>
This header declares functions for dealing with "old-fashioned"
C-strings.
- NULL
- The NULL macro expands to a constant null pointer whose
value is implementation-dependent, but usually 0 or 0L.
- size_t
- A typedef for an implementation-dependent signed
integral type which is the type returned by the sizeof
operator
- char* strcat(char* destination, const char* source)
- Add C-string source to end of C-string
destination and return destination
- char* strncat(char* destination, const char* source, size_t
n)
- Like strcat() above, but at most n characters
are concatenated
- int strcmp(const char* str1, const char* str2)
- Compare C-string str1 to C-string str2 and
return a value that is < 0, == 0, > 0 according as
str1 is alphabetically <, ==, or > str2
- int strncmp(const char* str1, const char* str2, size_t n)
- Like strcmp() above, but compares at most n
characters
- char* strcpy(char* destination, const char* source)
- Copy C-string source to C-string destination
and return destination
- char* strncpy(char* destination, const char* source, size_t
n)
- Like strcpy() above, but copies at most n
characters
- size_t strlen(const char* str)
- Return length of C-string str
- const char* strchr(const char* str, int ch)
char* strchr( char* str, int ch)
- Return address of first location in str where
ch found, or the NULL pointer if ch is not
found
- const char* strrchr(const char* str, int ch)
char* strrchr( char* str, int ch)
- Return address of last (rightmost) location in str where
ch found, or the NULL pointer if ch is not
found
- const char* strstr(const char* str, const char* substr)
char* strstr( char* str, const char* substr)
- Return address of first location in str where substring
substr found, or the NULL pointer if
substr is not found
- const char* strpbrk(const char* str, const char* span_set)
char* strpbrk( char* str, const char* span_set)
- Search str for any of the characters in
span_set, and return a pointer to the first occurrence of
such a character, or a NULL pointer if there is no such
character in span_set
- size_t strspn(const char* str, const char* span_set)
- Return the number of characters at the start of C-string
str that are in C-string span_set
- size_t strcspn(const char* str, const char* span_set)
- Return the number of characters at the start of C-string
str that are not in C-string span_set
- char* strtok(char* str, const char* delim_set)
- Split str into separate tokens, separated by one or more
characters from delim_set, returning the most recent token
found, or a NULL pointer if no token found [To parse a
string str, you must call strtok() multiple times,
and the contents of str are modified when each token is
found. The first time, pass str as the first parameter to
strtok(); in the second and subsequent calls pass, pass a
NULL pointer as the first parameter.]
<ctime>
This header declares types and functions for dealing with dates and
times.
- NULL
- The NULL macro expands to a constant null pointer whose
value is implementation-dependent, but usually 0 or 0L.
- size_t
- A typedef for an implementation-dependent signed
integral type which is the type returned by the sizeof
operator
- int CLOCKS_PER_SEC
- The number of clock ticks returned by clock() in one second
- clock_t
- A typedef for an implementation-dependent integral type
(long, say) for the return value of a call to the clock() function
- time_t
- A typedef for an implementation-dependent integral type
(long, say) for the return value of a call to the time() function
- tm
-
A structure for storing parts of a date and time, which is defined
as follows:
struct tm
{
int tm_sec; /* Seconds: 0-59 */
int tm_min; /* Minutes: 0-59 */
int tm_hour; /* Hours: 0-23 */
int tm_mday; /* Day of the month: 1-31 */
int tm_mon; /* Month: 0-11 */
int tm_year; /* Years since 1900 */
int tm_wday; /* Days since Sunday: 0-6 */
int tm_yday; /* Days since January 1: 0-365 */
int tm_isdst; /* Daylight Savings Time */
};
- clock_t clock()
- Return number of clock ticks of elapsed processor time since
program startup
- time_t time(time_t* time_t_ptr)
- Return current date and time in an implementation-defined format,
and if time_t_ptr is not NULL, store the return
value in *time_t_ptr as well) [So, the function call
time(NULL) will return the current date and time in the
time_t format.]
- char* asctime(const tm* tm_ptr)
- Formulate the date and time pointed to by tm_ptr as a
C-string and return that string [For example, a function call like
asctime(localtime(&t)) would
return current time/date string like Tue Sep
04 11:56:32 2001, provided t has been assigned
the result of a call to time(NULL).]
- char* ctime(const time_t* time_t_ptr)
- Convert the time pointed to by time_t_ptr to local time,
format it as a C-string and return that string [equivalent to asctime(localtime(time_t_ptr))]
- double difftime(time_t t1 time_t t2)
- Return the difference between the two times t1 and
t2 (t1-t2, in seconds) [Note the order of
subtraction.]
- tm* localtime(const time_t* time_t_ptr)
- Expand the calendar time pointed to by time_t_ptr into a
static tm using local time and return a pointer to the
static object
- time_t mktime(tm* tm_ptr)
- Make a time_t time by assembling the parts in a
tm object, interpreted as a local time, and return that
time_t time
<cwchar>
This header declares types and functions for working with wide
characters. The facilities provided by this header will not normally be
required in those situations and locales where the standard ASCII
character code set is adequate for most programming purposes.
<cwctype>
This header declares types and functions for classifying and
converting wide characters. The facilities provided by this header will
not normally be required in those situations and locales where the
standard ASCII character code set is adequate for most programming
purposes.
The 20 Specifically C++ Headers
These headers may be classified into the following three groups: ten
"stream-related" headers, four "language support" headers, and six
"odds and ends" headers.
The 10 "stream-related" headers
For more detailed information on C++ I/O in general, and to see how
the material in this subsection on the stream headers fits into the
overall scheme of things, look here.
<fstream>
This header declares some classes and other types for performing I/O
with external files, as well as member functions for opening and
closing files and testing whether a file is open. Under this header,
class names are shown as they would appear in a typical object
declaration, and member functions are presented in "typical usage"
format.
- fstream file;
- Declare an fstream object file that can be used
for reading input and/or writing output
- fstream file(c_string_value);
- Declare an fstream object file, connect it to
the physical file whose name is in the C-string
c_string_value, and open it for reading input and/or writing
output
- fstream file(c_string_value, openmode_value);
- Declare an fstream object file, connect it to
the physical file whose name is in the C-string
c_string_value, and open it using the specific value of type
openmode specified in openmode_value [See under
<ios> for the definition of the openmode
type.]
- ifstream inFile;
- Declare an ifstream object inFile that can be
used for reading input
- ifstream inFile(c_string_value);
- Declare an ifstream object inFile, connect it
to the physical file whose name is in the C-string
c_string_value, and open it for reading input
- ifstream inFile(c_string_value, openmode_value);
- Declare an ifstream object inFile, connect it
to the physical file whose name is in the C-string
c_string_value, and open it using the specific value of type
openmode specified in openmode_value [See under
<ios> for the definition of the openmode
type.]
- ofstream outFile;
- Declare an ofstream object outFile that can be
used for writing output
- ofstream outFile(c_string_value);
- Declare an ofstream object outFile, connect it
to the physical file whose name is in the C-string
c_string_value, and open it for writing output
- ofstream outFile(c_string_value, openmode_value);
- Declare an ofstream object outFile, connect it
to the physical file whose name is in the C-string
c_string_value, and open it using the specific value of type
openmode specified in openmode_value [See under
<ios> for the definition of the openmode
type.]
In each of the three groupings immediately above, the first form
just declares a file stream object, while the other two both declare a
file stream object and open a physical file attached to that object.
However, if the first form is used to declare a file stream object,
then the actual file has to be opened later via a call to the open
function, which is what the two forms shown below provide.
- anyFileStream.open(c_string_value)
- Connect the file stream object anyFileStream with the
physical file whose name is in the C-string c_string_value,
open it in a default mode which will depend on the actual class type
of anyFileStream, and return the this pointer if successful
or a NULL pointer if unsuccessful
- anyFileStream.open(c_string_value, openmode_value)
- Connect the file stream object anyFileStream with the
physical file whose name is in the C-string c_string_value,
open it in a mode determined by openmode_value, and return
the this pointer if successful or a NULL pointer if
unsuccessful [See under <ios> for the definition of
the openmode type.]
- anyFileStream.is_open()
- Return true if the file stream object
anyFileStream is open, and otherwise false
- anyFileStream.close()
- Close the physical file associated with the file stream object
anyFileStream, and disconnect the two
<iomanip>
This header declares the I/O manipulators that take parameters. They
are presented in "typical usage" format.
The following three manipulators provide one mechanism for setting
(or "unsetting") the format-state flags. There are member functions
under <ios> that accomplish the same purpose.
- setbase(int_value)
- Set the base (or conversion radix) for a stream to
int_value, which must be one of the following values, since
any other value will be interpreted as 0: 10 (for decimal), 8 (for
octal), or 16 (for hex)
- setiosflags(fmtflags_value)
- Set the format-state flags specified explicitly in
fmtflags_value and leave the remaining flags unchanged
(equivalent to stream.setf(fmtflags_value) under
<ios>) [See <ios> as well for the
definition of the fmtflags type.]
- resetiosflags(fmtflags_value)
- Clear the format-state flags specified explicitly in
fmtflags_value and leave the remaining flags unchanged
(equivalent to stream.unsetf(fmtflags_value) under
<ios>) [See <ios> as well for the
definition of the fmtflags type.]
The next three manipulators provide one mechanism for setting the
format-state parameters. There are member functions under
<ios> that accomplish the same purpose.
- setfill(int_value)
- Set the "fill" format-state parameter that determines the "fill
character" when numerical values are output to the character whose
internal integer code is int_value
- setprecision(int_value)
- Set the "precision" format-state parameter to int_value
places for floating-point values
- setw(int_value)
- Set the "width" format-state parameter to int_value
<ios>
This header declares the classes, types, and manipulators that form
the foundation of the C++ I/O library. A class called ios_base
is the base class for all I/O stream classes, and a class template
called basic_ios derives from ios_base and declares
the behavior that is common to all I/O streams.
All manipulators and member functions in this subsection are
presented in "typical usage" format.
The types in the following list are referred to in various places
later under this header and elsewhere on this web page. When you
encounter such a reference (as the type of a function parameter, say),
you may want to look back here at one of these type definitions to
verify that a parameter of that type makes sense for that function (for
example).
This list gives only a high-level description of each
typedef, which is further constrained by the fact that each
actual type is implementation-defined. For further information, check
this site's page on C++
Input/Output. The ellipsis (...) that appears in each list item is
the placeholder for the actual type name.
- fmtflags
- An implementation-defined integer, enumerated, or bitmask type
that represents formatting flags for I/O [Constant values of this
type are: boolalpha, dec, fixed,
hex, internal, left, oct,
right, scientific, showbase,
showpoint, showpos, skipws,
unitbuf, uppercase, adjustfield,
basefield, floatfield]
- iostate
- An implementation-defined integer, enumerated, or bitmask type
that represents the status (error state, if you like) of an I/O
stream [Constant values of this type are: badbit,
eofbit, failbit, goodbit =
iostate(0)]
- openmode
- An implementation-defined integer, enumerated, or bitmask type
that defines the mode for opening a file [Constant values of this
type are: app, ate, binary, in,
out, trunc]
- seekdir
- An implementation-defined enumerated type that specifies the
origin for seeking (moving the "read pointer" or "write pointer") to
a new file position [Constant values of this type are: beg,
cur, end]
- streamoff
- An implementation-defined type that represents a signed offset in
a stream [Values of type streammoff may be converted to
values of type streamsize without losing information.]
- streamsize
- An implementation-defined signed integral type used to represent
the size of various stream entities, such as the number of characters
to read or write in an I/O operation [Values of type
streamsize may be converted to values of type
streamoff without losing information.]
The following is a list of parameterless manipulators available from
this header.
- left
- Left-justify all output
- right
- Right-justify all output
- internal
- Left-justify sign or base indicator, and right-justify rest of
number
- dec
- Display integers in base 10 (decimal) format
- oct
- Display integers in base 8 (octal) format
- hex
- Display integers in base 16 (hexadecimal) format
- fixed
- Use fixed point notation when displaying floating-point numbers
(the usual convention)
- scientific
- Use exponential (i.e., scientific) notation when displaying
floating-point numbers
- showbase
noshowbase
- Show (or don't show) base indicator when displaying integer
output
- showpoint
noshowpoint
- Show (or don't show) decimal point when displaying floating-point
numbers (default is 6 significant digits)
- showpos
noshowpos
- Show (or don't show) a leading plus sign (+) when displaying
positive numbers
- uppercase
nouppercase
- Use (or don't use) uppercase E when displaying
exponential numbers, and uppercase letters when displaying
hexadecimal numbers
- boolalpha
noboolalpha
- Use (or don't use) textual rather than numerical format to read
and write boolean values (i.e., use, or don't use, true and
false rather than 1 and 0)
- skipws
noskipws
- Skip (or don't skip) whitespace on input
- unitbuf
nounitbuf
- Flush (or don't flush) output buffer after each insertion (which
really has nothing to do with formatting)
- stream.clear()
- Clear all stream error flags
- stream.good()
- Return true if no error has occurred (i.e., the next
three functions all return false)
- stream.eof()
- Return true if end-of-file has been encountered while
attempting an input operation
- stream.fail()
- Return true if an input or output operation failed
- stream.bad()
- Return true if input or output error was so severe that
recovery is unlikely
- stream.flags()
- Return a value of type fmtflags that contains the
current values of all format-state flags
- stream.flags(fmtflags_value)
- Return a value of type fmtflags that contains the
current values of all format-state flags, set all flags in
fmtflags_value, and clear all flags not mentioned in
fmtflags_value
- stream.setf(fmtflags_value)
- Set format-state flags specified in fmtflags_value,
leave all other format-state flags unchanged, and return a value of
type fmtflags that contains the previous state of all
flags
- stream.setf(fmtflags_value, fmtflags_group)
-
Set format-state flags specified in fmtflags_value as the
new flags for the flag group identified by fmtflags_group,
leave all other format-state flags unchanged, and return a value of
type fmtflags that contains the previous state of all
flags
Typical uses of the two-parameter version of this function look
like this:
stream.setf(ios::left, ios::adjustfield)
stream.setf(0, ios::floatfield)
That last version clears all flags in the
ios::floatfield bit field.
- stream.unsetf(fmtflags_value)
- Clear format-state flags specified in fmtflags_value and
leave all other format-state flags unchanged
- stream.fill()
- Return current fill character format-state parameter value as a
value of type char
- stream.fill(char_value)
- Return current fill character format-state parameter value as a
value of type char, and set fill character format-state
parameter to new value in char_value
- stream.precision()
- Return current precision format-state parameter value as a value
of type streamsize
- stream.precision(streamsize_value)
- Return current precision format-state parameter value as a value
of type streamsize, and set precision format-state parameter
to new value in streamsize_value
- stream.width()
- Return current fieldwidth format-state parameter value as a value
of type streamsize
- stream.width(streamsize_value)
- Return current fieldwidth format-state parameter value as a value
of type streamsize, and set fieldwidth format-state
parameter to new value in streamsize_value
<iosfwd>
This header provides forward declarations of the various I/O-related
classes and templates, but can be ignored by most programmers most of
the time. In any case, this header is included by
<ios>.
<iostream>
This header declares the following four "standard stream objects".
They are all initialized and "set up for business" before the main
program begins, and are not destroyed during normal program execution,
so they can be used throughout the life of a program. You can tell from
the class of each object which member functions are available for use
with that object by checking the class type of the object and looking
under the header containing that class heading elsewhere in this
subsection of the current web page.
- extern istream cin
- The "standard input stream"
- extern ostream cout
- The "standard output stream"
- extern ostream cerr
- The "standard error stream"
- extern ostream clog
- The "standard log stream"
<istream>
This header declares the input stream classes and templates, and one
input manipulator. In addition to the member functions given below, the
extraction operator, operator>>, is of course overloaded
to permit reading primitive values into variables, as well as the
reading of characters into C-style string variables and C++ string
objects.
- istream inStream;
- Declare an istream object that can be used for reading
input
- iostream ioStream;
- Declare an istream object that can be used for reading
input and/or writing output
- ws
- A manipulator that causes whitespace characters to be
skipped
- inStream.peek()
- Return integer code of next character to be read without changing
stream position (i.e., without actually reading the character and
removing it from the stream), or return the integer code for
end-of-file if there is no such character
- inStream.ignore()
- Ignore the next character in the stream, if there is one, and
return *this
- inStream.ignore(positive_integer_value)
- Ignore positive_integer_value characters, or all
characters up to the end-of-file, whichever comes first, and return
*this
- inStream.ignore(positive_integer_value,
char_delimiter_value)
- Ignore positive_integer_value characters, or all
characters up to, and including, the character in
character_delimiter_value, whichever comes first, and return
*this [The most generally useful form of this function looks
something like this: inStream.ignore(80,
'\n'), which is often used to ignore everything on the
rest of a line of input.]
- inStream.get()
- Read and return the integer code of the next single character
from inStream, or return the integer code for end-of-file if
there are no more characters to be read
- inStream.get(char_variable)
- Read the next single character from inStream into
char_variable, and return *this
- inStream.putback(char_value)
- Try to put the character in char_value back into
inStream so that it will be the next character read from the
input stream, and return *this
- inStream.unget(char_value)
- Same as inStream.putback(char_value) (see immediately
above)
In the following list, note that the difference between
get() and getline() is simply that get()
leaves the delimiter in the input stream, while getline()
removes it. Also, a technical point that can cause some confusion if it
actually occurs, and should thus be noted, is this: In the case of
getline(), if the maximum number of characters to be read is
exactly the same as the number of characters available before the
delimiter, then the delimiter is not actually "encountered", and is
therefore left in the input stream on such an occasion. The +1
in the n+1 parameter value can be thought of as an allowance
for the extra space to accommodate the terminating null character
('\0') that the C-style string variable (into which the
characters are being read) must contain.
- inStream.get(c_string_variable, n+1)
- Read up to n characters (or up to the newline character,
whichever comes first) into c_string_variable
- inStream.get(c_string_variable, n+1, char_delimiter_value)
- Read up to n characters (or up to the first occurrence
of char_delimiter_value, whichever comes first) into
c_string_variable
- inStream.getline(c_string_variable, n+1)
- Read up to n characters (or up to the newline character,
whichever comes first) into c_string_variable
- inStream.getline(c_string_variable, n+1,
char_delimiter_value)
- Read up to n characters (or up to the first occurrence
of char_delimiter_value, whichever comes first) into
c_string_variable
- inStream.read(c_string_variable, streamsize_value)
- Read up to streamsize_value characters, place them in
c_string_variable, and return *this [Note that no
null character is appended to c_string_variable.]
- inStream.gcount()
- Return the number of characters returned from the stream by the
most recent call to an unformatted member function (get(),
getline(), ignore(), peek(),
putback(), read() or unget())
- inStream.tellg()
- Return current position in an input stream as a value of type
streamoff [The name tellg is short for "tell get",
i.e., "tell" the position at which you may "get" a value.]
- inStream.seekg(streamoff_value)
- Move the "read pointer" in an input stream to the explicit
position, or "offset" (measured by default from the beginning of the
stream) specified by streamoff_value [The name
seekg is short for "seek get", i.e., "seek a (new) position
at which you may "get" a value.]
- inStream.seekg(streamoff_value, seekdir_value)
- Move the "read pointer" in an input stream in a direction
determined by seekdir_value and a distance specified by
streamoff_value
<ostream>
This header declares the output stream class templates,
specializations and manipulators. See <fstream> for
derived classes that write to files, <sstream> for
derived classes that write to strings, and <ios> for
base-class declarations. In addition to the member functions given
below, the insertion operator, operator<<, is of course
overloaded to permit output of primitive values, as well as C-style
strings and C++ string objects.
- ostream outStream;
- Declare an ostream object that can be used for writing
output
- endl
- Insert newline and flush the output stream
- ends
- Insert a null character
- flush
- Flush an output stream
- outStream.flush()
- Flush the output buffer and return *this
- outStream.put(char_value)
- Write the character in char_value to the stream and
return *this
- outStream.write(c_string_value, streamsize_value)
- Write streamsize_value characters from
c_string_value to the output stream and return
*this
- outStream.tellp()
- Return current position in an output stream as a value of type
streamoff [The name tellp is short for "tell put",
i.e., "tell the position at which you may "put", or write, a
value.]
- outStream.seekp(streamoff_value)
- Move the "write pointer" in an output stream to the explicit
position, or "offset" (measured by default from the beginning of the
stream) specified by streamoff_value [The name
seekp is short for "seek put", i.e., "seek" a (new) position
at which you may "put", or write, a value.]
- outStream.seekp(streamoff_value, seekdir_value)
- Move the "write pointer" in an output stream in a direction
determined by seekdir_value and a distance specified by
streamoff_value
<sstream>
This header declares classes, templates and other types for reading
from and writing to string objects in memory in the same manner as
reading from and writing to files.
The following declarations parallel the analogous declarations for
file streams under <fstream>. In each case a string
buffer is created in memory, which can then be used for input and/or
output, as the case may be.
- istringstream inStringStream;
- Declare an istringstream object which is initially
empty, but which can be used for input (after something is placed in
it, of course)
- istringstream inStringStream(string_variable);
- Declare an istringstream object which has its buffer
initialized with the contents of string_variable and which
can be used for input
- ostringstream outStringStream;
- Declare an ostringstream object which is initially
empty, but which can be used for output
- ostringstream outStringStream(string_variable);
- Declare an ostringstream object which has its buffer
initialized with the contents of string_variable and which
can be used for output
- stringstream ioStringStream;
- Declare a stringstream object which is initially empty,
but which can be used for either input or output
- stringstream ioStringStream(string_variable);
- Declare a stringstream object which has its buffer
initialized with the contents of string_variable and which
can be used for either input or output
- anyStringStream.str()
- Return the contents of the string buffer associated with the
stream as a string value
- anyStringStream.str(string_variable)
- Deallocate the current buffer and replace it with the contents of
string_variable
<streambuf>
This header declares the basic_streambuf class template and
its streambuf specialization, which provides a stream buffer
object to manage low-level access to a sequence of characters. This
header is not normally needed in day-to-day programming.
<strstream>
This header declares classes, templates and other types for reading
from, and writing to, character arrays in memory, in the same manner as
reading from and writing to files. This header and its classes are
actually deprecated in the Standard, another hint that programmers
should really be using C++ string objects and the facilities from
<sstream> instead.
The 4 "language support" headers
<exception>
This header declares classes, types and functions related to
fundamental exception handling. See header <stdexcept>
for additional exception classes. That header, rather than this one,
will be the one most programmers will want to include if they wish to
make use of the standard exceptions.
The following diagram shows both the C++ exception hierarchy, and
(since the majority of exception classes are not found in the
current header) the header in which each class is found.
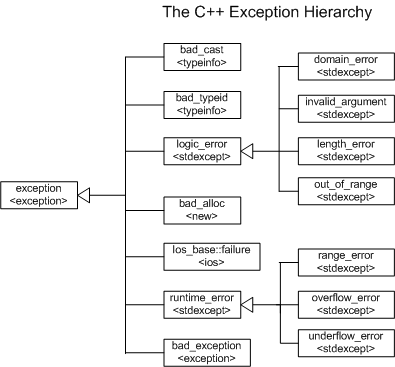
- anyStandardException.what()
- Return an implementation-defined message as a C-string value
<new>
This header declares types and functions related to dynamic memory
management. However, most programmers will not need this header, since
the built-in new and delete operators are sufficient for most purposes,
and the facilities provided by this header deal more with custom
management of dynamic memory, such as writing one's own versions of new
and delete.
<stdexcept>
This header defines several standard exception classes, though the
base exception class is found in <exception>. Note that
the Standard Library itself does not have many places where exceptions
are thrown. The exception classes provided in the current header, and
several other headers, are for the average programmer's use, should one
of the library-provided exception classes, or an exception class
derived from one of those classes, be found useful. See the
hierarchical diagram in <exception> to see which headers
contain which classes.
<typeinfo>
This header declares the type_info class (for the
typeid operator) and two exception classes related to type
information and casting.
- type_info
- This is a class name. When using RTTI (Run-Time Type
Identification) and the typeid operator, programmers need to
know that the typeid operator returns an object of this
class type. Such objects have a name() member function which
returns the name of the object (the name of a type) as a C-string
value. It is also helpful to know that operator== and
operator!= are overloaded for such objects as well.
The 6 "odds and ends" headers
<bitset>
This header declares a single class called bitset, and some
related functions. A bitset object is a fixed-size sequence of
bits, for which the usual bitwise operators are overloaded to work in
the usual way. You can also access individual bits by index.
More to come ...
<complex>
This header declares the complex class template and
specializations to work with float, double and
long double component types, as well as mathematical functions
that work with complex values.
More to come ...
<limits>
This header declares the numeric_limits class template and
related types and specializations that define the limits and
characteristics of the fundamental arithmetic types. It may be viewed
as the C++ equivalent of the two legacy C headers
<cfloat> and <climits>.
More to come ...
<locale>
This header declares class and function templates that support
internationalization and localization. Most programmers can simply
ignore this header, and accept the local system defaults.
<memory>
This header declares functions and class templates for allocating
and using memory. The average programmer will not normally find it
necessary to include this header.
However, those programmers who are developing their own
STL-compatible containers, iterators and algorithms may find the header
useful. Every standard STL container and adaptor class needs an
allocator to provide the necessary memory, but the default
allocator provided in each case is nearly always the one to
use, and this is provided for the client programmer automatically in
each case when an object of a given class is declared.
The <memory> header also provides the
auto_ptr class. This class gives us a kind of "smart pointer"
that helps to avoid memory leaks when exceptions are thrown, but a
great deal of C++ programming can be done without resorting to the
useof auto_ptr.
<string>
This header declares the class templates and functions that support
the string type, which is one specialization of the basic_string class
template. For most purposes, C++ string objects should be preferred
over "old-fashioned" C-style strings because of their added flexibility
and safety. Constructors and functions are given in "typical usage"
format.
- size_type
- An unsigned integral type for string size values and
string indices (equivalent to size_t)
- npos
- Class static constant value, of type string::size_type,
initialized by -1, and meaning either "not found" (as the return
value of a search function, for example), or "all remaining
characters" (as in an erase function, for example) [Be careful to use
the proper type for such a value, and not, for example, an
int value.]
The following declaration statements illustrate string
constructors.
- string s;
- Construct an empty string object s
- string s(c_string_value);
- Construct a string object s from the C-string
in c_string_value
- string s(char_array, size_type_count);
- Construct a string object s, initialized by the
first size_type_count characters from the character array
char_array [Note: char_array might, in fact, be a
C-string, but, as its name suggests, it might also be just an
ordinary array of characters.]
- string s(string_value);
- Construct a string object s as a copy of the
other string object in string_value
- string s(string_value, size_type_index);
- Construct a string object s initialized by the
characters in string_value from index
size_type_index in string_value to the end of
string_value
- string s(string_value, size_type_index, size_type_count);
- Construct a string object s initialized by, at
most, size_type_count characters from string_value,
starting at index size_type_index in
string_value
- string s(size_type_count, char_value);
- Construct a string object s consisting of
size_type_count copies of char_value
- string s(input_iterator_start, input_iterator_end);
- Construct a string object s initialized by all
characters from the input iterator range [input_iterator_start,input_iterator_end)
- s[i]
- Return a reference to the character at index i of
string object s [Indices of string objects
start at 0.]
- s.at(i)
- Same as s[i], but with out-of-bounds checking
The following are iterators that allow objects of the string class
to work with algorithms and containers of the STL, but may not be
needed for many everyday uses of C++ strings.
- s.begin()
- Return an iterator object that points to the first
character of the string object s
- s.end()
- Return an iterator object that points to "one character
position past the end" of the string object s
- s.rbegin()
- Return a reverse_iterator object that points to the last
character of the string object s
- s.rend()
- Return a reverse_iterator object that points to "one
position before the first character" of the string object
s
In addition to the following variations on the append()
member function, operator+= is also overloaded to append a C++
string object, a C-string, or a single character, to a C++
string object.
- s.append(string_value)
- Append string object in string_value to
s and return the revised s
- s.append(c_string_value)
- Append C-string in c_string_value to s and
return the revised s
- s.append(size_type_count, char_value)
- Append size_type_count copies of the character in
char_value to s and return the revised
s
- s.append(c_string_value, size_type_count)
- Append size_type_count characters from
c_string_value to s, and return the revised
s
- s.append(c_string_value, size_type_index, size_type_count)
- Append to s at most size_type_count characters
from c_string_value, starting at size_type_index,
and return the revised s
- s.append(first_input_iterator, last_input_iterator)
- Append to s all characters in the iterator
range [first_input_iterator,last_input_iterator)
and return the revised s
In addition to the following variations on the assign()
member function, operator= is also overloaded to assign a C++
string object, a C-string, or a single character, to a C++
string object.
- s.assign(string_value)
- Assign string object in string_value to
s and return the revised s
- s.assign(c_string_value)
- Assign C-string in c_string_value to s and
return the revised s
- s.assign(size_type_count, char_value)
- Assign size_type_count copies of the character in
char_value to s and return the revised
s
- s.assign(c_string_value, size_type_count)
- Assign size_type_count characters from
c_string_value to s, and return the revised
s
- s.assign(c_string_value, size_type_index, size_type_count)
- Assign to s at most size_type_count characters
from c_string_value, starting at size_type_index,
and return the revised s
- s.assign(start_input_iterator, end_input_iterator)
- Assign to s all characters in the iterator range [start_input_iterator,end_input_iterator)
and return the revised s
- s.copy(char_array, size_type_count, size_type_index)
- Copy up to size_type_count characters from s,
starting at index size_type_index, to the character array
char_array, and return the number of characters copied
(which will be the smaller of size_type_count and size()
- size_type_index)
- s.c_str()
- Return base address of a C-string (i.e., a null-terminated
character array) containing characters from s [Note that the
return value of this function is "owned" by s and should not
be altered.]
- s.data()
- Return base address of a character array (which is not
null-terminated) containing characters from s [Note that the
return value of this function is "owned" by s and should not
be altered.]
- s.substr(size_type_index)
- Return a copy of the substring of s consisting of those
characters starting at index size_type_index, and extending
to the end of s
- s.substr(size_type_index, size_type_count)
- Return a copy of the substring of s that starts at index
size_type_index and extends for up to
size_type_count characters (the smaller of
size_type_count and size() - size_type_index)
- s.empty()
- Return true if s contains no characters,
false otherwise
- s.capacity()
- Return number of characters alloted for use by the
string object s, as a value of type
string::size_type
- s.length()
- Return the number of characters in s (equivalent to
s.size())
- s.size()
- Return the number of characters (not the number of
bytes) in s (equivalent to s.length())
- s.max_size()
- Return the size of the largest possible string object
- s.reserve(size_type_value)
- Make capacity of s at least size_type_value
[This function is not normally needed, but might be useful if, for
example, you knew that a string was going to grow by small increments
to a large size and you wanted to avoid a large number of storage
reallocations.]
- s.resize(size_type_value, char_value)
- Change size of s to size_type_value [If
size_type_value <= size(), the new s will
contain the first size_type_value characters of the old
s. If size_type_value > size(), the new
s is the same as the old s, but with
size_type_value-size() copies of the character in
char_value appended to its end.]
- s.resize(size_type_value)
- Same as the previous version of resize(), except that in
this case the character value appended is the default character value
(the null character)
- s.clear()
- Erase all characters in the string [This is a void
function, unlike erase() below.]
- s.erase()
- Erase all characters in the string, and return the empty string
object s
- s.erase(size_type_index)
- Remove all characters from index size_type_index onward,
and return the revised s
- s.erase(size_type_index, size_type_count)
- Remove size_type_count characters from s,
beginning at size_type_index, and return the revised
s [If size_type_count is too large, characters are
erased only to the end of s.]
- s.erase(iterator_position)
- Remove the character at the position specified by
iterator_position and return an iterator pointing at the
next character, if there is one, or the iterator object
end() if there isn't
- s.erase(first_iterator, last_iterator)
- Remove all characters in the iterator range specified by
[first_iterator,last_iterator)
and return an iterator pointing at the character that
last_iterator was pointing at before the deletion took
place, or the iterator object end() if
last_iterator was the iterator object
end()
The functions in this group search for a single character and return
the index where it is found, if it is, or the value
string::npos if it isn't.
- s.find(char_value)
s.find(char_value, size_type_index)
s.rfind(char_value)
s.rfind(char_value, size_type_index)
- The find() functions search forward, and return the
first index where the character is found. The rfind()
functions search backward, and return the last index where the
character is found. The search begins at the index specified by
size_type_index, if that parameter is present. Otherwise the
search starts at the beginning of the string (for find()) or
at the end of the string (for rfind()).
The functions in this group search for a substring in the target
string s (the first occurrence in the case of find(),
the last one in the case of rfind()), and return the index of
the first character of that substring if it is found, or the value
string::npos if it isn't.
- s.find(string_value)
s.find(string_value, size_type_index)
s.rfind(string_value)
s.rfind(string_value, size_type_index)
- The find() functions search forward, and the
rfind() functions search backward. The search begins at the
index specified by size_type_index, if that parameter is
present. Otherwise the search starts at the beginning of the string
(for find()) or at the end of the string (for
rfind()). [These functions are also overloaded to
work if the C++ string object string_value is replaced by a
C-string variable, say c_string_value.]
- s.find(c_string_value, size_type_index, size_type_count)
s.rfind(c_string_value, size_type_index, size_type_count)
- These functions search for size_type_count characters of
c_string_value in s. The find() function
searches forward, and the rfind() function searches
backward. The search begins at the index specified by
size_type_index, if that parameter is present. Otherwise the
search starts at the beginning of the string (for find()) or
at the end of the string (for rfind()).
The functions in this group search the target string s for
the first occurrence of a character that also appears/does not appear
in a specified group of one or more characters. They return the index
where the character has been found, or the value string::npos
if the search was unsuccessful.
- s.find_first_of(char_value)
s.find_first_of(char_value, size_type_index)
s.find_first_not_of(char_value)
s.find_first_not_of(char_value, size_type_index)
- These functions all search forward. The search begins at the
index specified by size_type_index, if that parameter is
present. Otherwise the search starts at the beginning of the
string.
- s.find_first_of(string_value)
s.find_first_of(string_value, size_type_index)
s.find_first_not_of(string_value)
s.find_first_not_of(string_value, size_type_index)
- These functions all search forward in s looking for a
character in/not in string_value. The search begins at the
index specified by size_type_index, if that parameter is
present. Otherwise the search starts at the beginning of the string.
[These functions are also overloaded to work if the C++
string object string_value is replaced by a
C-string variable, say c_string_value.]
- s.find_first_of(c_string_value, size_type_index, size_type_count)
s.find_first_not_of(string_value, size_type_index,
size_type_count)
- These functions all search forward in s looking for a
character in/not in the size_type_count characters of
c_string_value starting at size_type_index.
The functions in this group search the target string s for
the last occurrence of a character that also appears/does not appear in
a specified group of one or more characters. They return the index
where the character has been found, or the value string::npos
if the search was unsuccessful.
- s.find_last_of(char_value)
s.find_last_of(char_value, size_type_index)
s.find_last_not_of(char_value)
s.find_last_not_of(char_value, size_type_index)
- These functions all search backward. The search begins at the
index specified by size_type_index, if that parameter is
present. Otherwise the search starts at the end of the string.
- s.find_last_of(string_value)
s.find_last_of(string_value, size_type_index)
s.find_last_not_of(string_value)
s.find_last_not_of(string_value, size_type_index)
- These functions all search backward in s looking for a
character in/not in string_value. The search begins at the
index specified by size_type_index, if that parameter is
present. Otherwise the search starts at the end of the string.
[These functions are also overloaded to work if the C++
string object string_value is replaced by a
C-string variable, say c_string_value.]
- s.find_last_of(c_string_value, size_type_index, size_type_count)
s.find_last_not_of(string_value, size_type_index,
size_type_count)
- These functions all search backward in s looking for a
character in/not in the size_type_count characters of
c_string_value starting at size_type_index.
The functions in this group insert one or more characters into the
target string s.
- s.insert(size_type_index, string_variable)
s.insert(size_type_index, c_string_value)
- Insert the characters from string_variable (or
c_string_value) into s so that those new characters
start at index size_type_index, and return
*this
- s.insert(size_type_index1, string_variable,
size_type_index2, size_type_count)
- Insert at most size_type_count characters from
string_variable, into s, starting at
size_type_index2 of string_variable, so that the
new characters in s start at index size_type_index1 of
s, and return *this
- s.insert(size_type_index, c_string_value, size_type_count)
- Insert size_type_count characters from
c_string_value into s so that the new characters
start at index size_type_index in s, and return
*this [The parameter c_string_value must contain at
least size_type_count characters, and the '\0'
character has no special meaning.]
- s.insert(size_type_index, size_type_count, char_value)
- Insert size_type_count copies of char_value
into s so that they start at index size_type_index
in s, and return *this
- s.insert(iterator_position, size_type_count, char_value)
- Insert size_type_count copies of char_value
into s before the character pointed to by
iterator_position, and return *this [Note that,
unlike the version of insert() immediately above, this is a
void function.]
- s.insert(iterator_position, char_value)
- Insert a copy of char_value into s before the
character pointed to by iterator_position, and return an
iterator object pointing to the inserted character
- s.insert(iterator_position, input_iterator_first,
input_iterator_last)
- Insert all characters from the range [input_iterator_first,input_iterator_last)
into s before the character pointed to by
iterator_position [Note that, unlike the version of
insert() immediately above, this is a void
function.]
- s.push_back(char_value)
- Append a copy of char_value to s
The functions in this group replace one or more characters in the
target string s.
- s.replace(size_type_index, size_type_count, string_value)
- Replace at most size_type_count characters of
s, starting at index size_type_index in s,
with all characters in string_value, and return
*this
- s.replace(iterator_first, iterator_last, string_value
- Replace all characters of s in the range [iterator_first, iterator_last), with
all characters in string_value, and return
*this
- s.replace(size_type_index1, size_type_count1, string_value,
size_type_index2, size_type_count2)
- Replace at most size_type_count1 characters of
s, starting at size_type_index1 of s, with
at most size_type_count2 characters from
string_value, starting at size_type_index2 in
string_value
- s.replace(size_type_index, size_type_count, c_string_value)
- Replace at most size_type_count characters of
s, starting at index size_type_index in s,
with all characters from c_string_value
- s.replace(iterator_first, iterator_last, c_string_value)
- Replace all characters of s in the range [iterator_first,iterator_last) with
all characters from c_string_value
- s.replace(size_type_index, size_type_count1,
c_string_value, size_type_count2)
- Replace at most size_type_count1 characters of
s, starting at size_type_index in s, with
size_type_count2 characters from
c_string_value
- s.replace(iterator_first, iterator_last,
c_string_value, size_type_count)
- Replace all characters from s in the range [iterator_first,iterator_last) with
size_type_count characters from c_string_value [The
parameter c_string_value must contain at least
size_type_count characters, and the '\0' character
has no special meaning.]
- s.replace(size_type_index, size_type_count1,
size_type_count2, char_value)
- Replace at most size_type_count1 characters of
s, starting at index size_type_index in s,
with size_type_count2 copies of char_value
- s.replace(iterator_first, iterator_last,
size_type_count, char_value)
- Replace all characters of s in the range [iterator_first, iterator_last) with
size_type_count copies of char_value
- s.replace(iterator_first, iterator_last,
input_iterator_start, input_iterator_end)
- Replace all characters of s in the range [iterator_first, iterator_last) with
all characters in the range [input_iterator_start,input_iterator_end)
The usual relational operators (==, !=, <, > <=, and >=)
are all overloaded to work with C++ string objects, and
perform lexicographic comparisons. The functions in the following group
also perform lexicographic comparisons of various kinds. In each case,
the return value is a value of type int, which is determined
as follows:
- a value < 0 if s is < the value with which it is
being compared
- a value > 0 if s is > the value with which it is
being compared
- 0 if s and the value with which it is being compared are
the same
- s.compare(string_value)
- Compare the characters in s with the characters in
string_value
- s.compare(size_type_index, size_type_count, string_value)
- Compare the characters in s with at most
size_type_count characters from string_value,
starting at index size_type_count of
string_value
- s.compare(size_type_index1, size_type_count1, string_value,
size_type_index2, size_type_count2)
- Compare at most size_type_count1 characters of
s, starting at index size_type_index1 of s
with at most size_type_count2 characters of
string_value, starting at size_type_index1 of
s
- s.compare(c_string_value)
- Compare the characters in s with the characters in
c_string_value
- s.compare(size_type_index, size_type_count, c_string_value)
- Compare at most size_type_count characters of
s, starting at index size_type_index of s,
with all characters of c_string_value
- s.compare(size_type_index, size_type_count1,
c_string_value, size_type_count2)
- Compare at most size_type_count1 characters of
s, starting at index size_type_index of s,
with size_type_count characters of c_string_value
[The parameter c_string_value must contain at least
size_type_count2 characters, and the '\0' character
has no special meaning.]
- s.swap(string_variable)
- Swap contents of s and string_variable
Note that the following three functions are free functions,
i.e., they are not member functions of the string class.
- swap(string_variable1, string_variable2)
- Swap contents of string_variable1 and
string_variable2
- getline(inStream, string_variable)
- Read a line of text into string_variable from
inStream and remove the newline from inStream
- getline(inStream, string_variable, char_delimiter_value)
- Read a line of text up to (but not including)
char_delimiter_value into string_variable, from
inStream, and remove char_delimiter_value from
inStream
<valarray>
This header declares types and functions for operating on arrays of
numerical values. Though the idea was that these operations would be
optimized, and thus very fast, C++ programmer consensus seems to be
that this part of the Standard Library has failed to live up to
expectations. In any case, most programmers will not need to use
<valarray>.
The 11 Standard Template Library (STL)
Headers
Only the names of the STL headers, and a very brief description of
the contents of each, are given on this page, in the categorized list
that appears immediately below. For more detailed information on the
STL, look here.
Headers for the STL "sequence containers"
<deque>
This header provides a double-ended queue container, with fast
insertion and deletion at both ends and (somewhat counterintuitively to
the abstract notion of queueness) direct access to any element.
<list>
This header provides a sequence container with fast performance when
adding elements to, or removing elements from, any point in the
sequence, but with only sequential access to any particular
element.
<vector>
This header provides the vector container, which is best thought of
as a generalized array, capable of "growing and shrinking" as the
occasion demands. Vectors provide fast insertion and deletion at one
end, and direct access to any element.
Headers for the STL "associative containers"
<map>
This header provides map and multimap classes which store key-value
pairs. The map requires unique keys, but the multimap permits duplicate
keys.
<set>
This header provides set and multiset classes which store keys. The
set can store only unique keys, but the multiset permits duplicate
keys.
Headers for the STL "container adaptors"
<queue>
This header provides containers which are actually "adapted"
sequential containers and provide the usual FIFO behavior of a standard
queue structure, as well as "priority queue" behavior.
<stack>
This header provides a container which is actually one of the above
containers "adapted" to provide the usual LIFO behavior of a stack
structure.
Headers for the STL algorithms
<algorithm>
This header provides a large number (about 70, depending on how you
count) generic algorithm function templates for operating on iterators,
as well as some other objects such as "function objects" that help
algorithms to perform their tasks. It is part of the genius, power and
flexibility of the STL that these algorithms do not operate on
containers directly, but on iterators that point to containers. This
means that under quite general conditions, the same algorithm can
operate on several different containers, and in a variety of ways,
which is, of course, the whole point of "generic programming".
<numeric>
This header declares a small number (four) of function templates
specifically for numerical algorithms.
Headers for the STL iterators and function objects
<iterator>
This header provides classes and templates for defining and using
iterators, though it does not have to be included if you are just using
one or more of the sequential and/or associative containers and
their associated iterators, since those iterators will be
available from the container classes themselves.
<functional>
This header defines several function objects. These may
also be called functionals, or functors. A function
object is an object of a class that implements operator(). This permits
the function object to be "called", using the same syntax as a
function, to help an algorithm perform its task. But, because it is an
object, it is more versatile than a function.
Miscellaneous STL headers
<memory>
This header declares functions and class templates for allocating
and using memory. The average programmer will not need to include this
header, since the default container "allocators" are perfectly adequate
most of the time. Programmers who are developing their own containers,
iterators and algorithms will be more inclined to find a use for what's
in this header.
<utility>
This header declares the pair<> template, which is
essential when using maps and multimaps, but also finds many uses in
everyday programming. Though it may or may not be of interest to the
average programmer, this header also defines the rel-ops namespace,
which in turn defines relational operators in terms of == and
<.